Arduino motion sensor
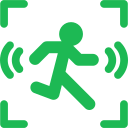
Last week , I decided to pick up an Arduino-based project — mainly because I missed dabbling in a project that was outside of my comfort zone. Plus, I hadn’t touched a bread-board or a soldering iron in years (6 years to be precise).
As all project ideas go, I turned to Pinterest 😅
Found a super-informative post by Angus Young (Gus), one of 2 maintainers of the PiMyLifeUp blog page. It gives you a really good starting point for an Arduino-virgin like me. I plan on doing my best to put into words, the steps I took, mistakes I made and what the end-product looked like!
Parts required:
- LED bulbs
- Jumper wires for the board
- 100 ohm resistors
- Piezo-buzzer (only get this if your partner doesn’t mind annoying sounds)
- Breadboard
- Adafruit PIR motion sensor
- Arduino (or Elegoo — the cheaper but functionally identical option) Uno R3 Board
Software required:
The only real software I needed was the Arduino IDE installed — this works for both the Arduino and Elegoo boards:

Code:
Here’s the code I used — a lot of it derived from Gus’ project I mentioned earlier.
Please ignore the pitches header being included — I was messing around with some melodies with the piezo-buzzer before my girlfriend walked by and unplugged it.
#include "pitches.h"
int ledPin = 4;
int piezoBuzzerPin = 3;
int pirSensorPin = 2;
int motionDetected = HIGH;
int blinkTime = 100;
int melody[] = {NOTE_C4, NOTE_G3, NOTE_G3, NOTE_A3, NOTE_G3, 0, NOTE_B3, NOTE_C4};
int noteDurations[] = {4, 8, 8, 4, 4, 4, 4, 4};
void setup() {
pinMode(ledPin, OUTPUT); // declare LED as output
pinMode(pirSensorPin, INPUT); // declare the PIR sensor as input
pinMode(piezoBuzzerPin, OUTPUT); //declare buzzer as output
Serial.begin(9600); //Set serial out if we want debugging
delay(1000); //Allow time for the PIR Sensor to callibrate
for (int thisNote = 0; thisNote < 8; thisNote++) {
int noteDuration = 1000 / noteDurations[thisNote];
tone(8, melody[thisNote], noteDuration);
// the note's duration + 30% seems to work well:
int pauseBetweenNotes = noteDuration * 1.30;
delay(pauseBetweenNotes);
noTone(8);
}
}
int counter=0;
void blinkNow(int repeats, int time)
{
for (int i = 0; i < repeats; i++)
{
digitalWrite(ledPin, HIGH);
digitalWrite(piezoBuzzerPin, HIGH);
delay(time);
digitalWrite(ledPin, LOW);
digitalWrite(piezoBuzzerPin, LOW);
delay(time);
int noteDuration = 1000 / noteDurations[0];
tone(8, melody[0], noteDuration);
}
}
void loop() {
delay(3000); //Setting this delay to give the PIR sensor some time to reset
motionDetected = digitalRead(pirSensorPin);
digitalWrite(ledPin, LOW);
digitalWrite(piezoBuzzerPin, LOW);
if (motionDetected == HIGH)
{
counter+=1;
Serial.print("Count = "); Serial.println(counter);
blinkNow(1, blinkTime);
}
}